|
[SDL] Couleur de la surface en fonction de sa position Difficulté : 10/100 |
Publié le 05/02/2008 à 19h 11min par Marco565
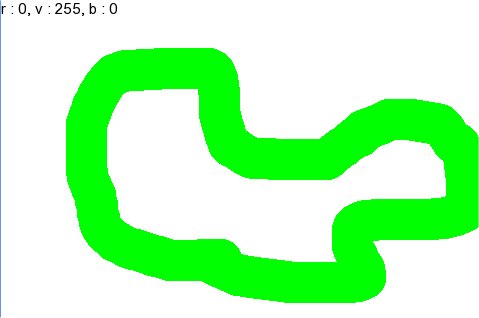 Afficher la couleur(R,V,B) en fonction de la position de la sourie sur la surface
Cet exercice requière une maîtrise de SDL.
Vous aurez besoin de la lib SDL_TTF et du SDK de SDL.
Cahier des charges :
afficher la couleur de la position a la quelle se trouve la sourie sur la surface (on utiliseras une image)
grâce a la fonction, qui se trouve dans la doc de SDL, nous allons récupéré la couleur de la positon de la sourie sur l'image.
pour ceux qui aurais la fleme d'aller chercher la fonction dans la doc je vous la donne :
Code : cUint32 getPixel(SDL_Surface *surface, int x, int y) { int bpp = surface->format->BytesPerPixel; /* Here p is the address to the pixel we want to retrieve */ Uint8 *p = (Uint8 *)surface->pixels y * surface->pitch x * bpp; switch(bpp) { case 1: return *p; case 2: return *(Uint16 *)p; case 3: if(SDL_BYTEORDER == SDL_BIG_ENDIAN) return p[0] << 16 | p[1] << 8 | p[2]; else return p[0] | p[1] << 8 | p[2] << 16; case 4: return *(Uint32 *)p; default: return 0; /* shouldn't happen, but avoids warnings */ } }
Aide :
la fonction renvoi un Uint32, il faut le separer en 3 Uint8 en fesant
Code : cUint32 couleur; Uint8 r, v, b; SDL_GetRGB(couleur, ecran->format, &r, &g, &b);
Correction
Vous n'y arrivez pas a trouver, ne vous découragez pas voici la correction:
Code : c#include <stdlib.h> #include <stdio.h> #include <SDL/SDL.h> #include <SDL/SDL_TTF.h> #include <SDL/SDL_image.h> Uint32 getPixel(SDL_Surface *surface, int x, int y); int main(int argc, char** argv) { SDL_Surface *ecran, *image, *texte; SDL_Rect pos; TTF_Font *police; SDL_Color noir = {0,0,0}; SDL_Init(SDL_INIT_VIDEO); TTF_Init(); ecran = SDL_SetVideoMode(480, 320, 32, SDL_HWSURFACE); image = SDL_LoadBMP("image.bmp"); pos.x = 0; pos.y = 0; char txt[100]; police = TTF_OpenFont("arial.ttf", 15); int continuer = 1; int mouseX = 0, mouseY = 0; Uint32 couleur; Uint8 r = 0, b = 0, g = 0; while(continuer) { SDL_Event event; SDL_WaitEvent(&event); switch(event.type) { case SDL_QUIT: continuer = 0; break; case SDL_MOUSEMOTION: mouseX = event.motion.x; mouseY = event.motion.y; break; } couleur = getPixel(image, mouseX, mouseY); SDL_GetRGB(couleur, ecran->format, &r, &g, &b); sprintf(txt, "r : %d, v : %d, b : %d", (int)r, (int)g, (int)b); texte = TTF_RenderText_Blended(police, txt, noir); SDL_FillRect(ecran, NULL, 0); SDL_BlitSurface(image, NULL, ecran, &pos); SDL_BlitSurface(texte, NULL, ecran, &pos); SDL_Flip(ecran); } SDL_FreeSurface(ecran); SDL_FreeSurface(image); TTF_CloseFont(police); TTF_Quit(); SDL_Quit(); return 0; } Uint32 getPixel(SDL_Surface *surface, int x, int y) { int bpp = surface->format->BytesPerPixel; /* Here p is the address to the pixel we want to retrieve */ Uint8 *p = (Uint8 *)surface->pixels + y * surface->pitch + x * bpp; switch(bpp) { case 1: return *p; case 2: return *(Uint16 *)p; case 3: if(SDL_BYTEORDER == SDL_BIG_ENDIAN) return p[0] << 16 | p[1] << 8 | p[2]; else return p[0] | p[1] << 8 | p[2] << 16; case 4: return *(Uint32 *)p; default: return 0; /* shouldn't happen, but avoids warnings */ } }
|
|